Coding standards: is consistency prettier than freedom?
Different projects have different coding standards, and some have none at all. How does it affect the quality of code and
developers' well-being? What results can we reasonably expect from a style guide?
Let's have a look at the effect of style guides in the real world. Here's how Jerusalem looks like:
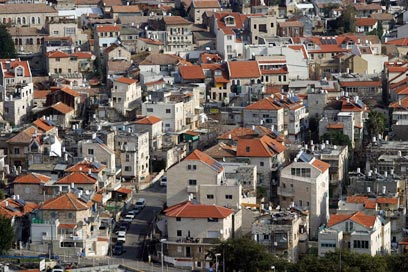
These similarly looking buildings are near the city center. Here's a shot of the suburbs:
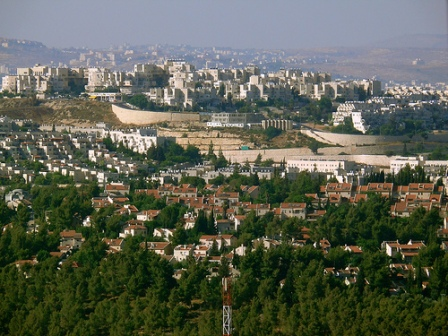
Same stuff, pretty much. White buildings, red roof tiles – or plain flat roofs.
And now for something completely different:
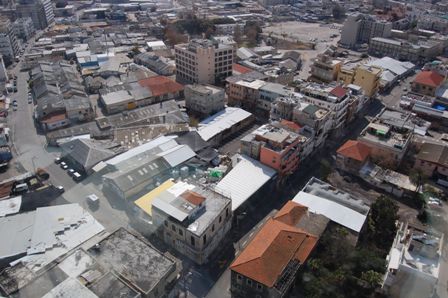
This is Tel Aviv. Buildings don't look similar to each other here. Nor do different parts of the city:
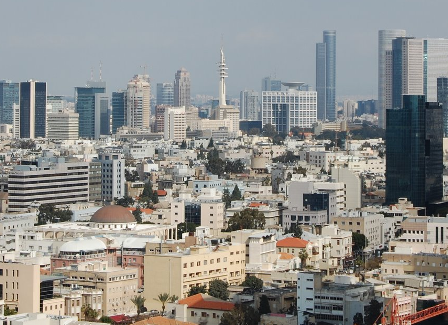
As you can see, in Tel Aviv, there's no style guide – everyone builds stuff to suit their own taste.
In Jerusalem, on the other hand, buildings have to be covered with Jerusalem stone, giving them their trademark off-white
color. Jerusalem owes its visual consistency to a
century-old style guide enforced by municipal laws.
Here are a few observations – relevant to most style guides, I think:
- Consistent style is either enforced or lacking. Whatever virtues freedom may have, consistency of style is
not one of them.
- Consistent style is functionally inconsequential. Buildings in Jerusalem are about as safe and comfortable
as buildings in Tel Aviv.
- Psychologically, style does matter. Many people hate visiting Tel Aviv because it's ugly.
- Whether consistent style is more beautiful is debatable. Many other people hate visiting Jerusalem
because it's ugly.
- People will defeat stylistic consistency despite the style guide. Here's an example from one of Jerusalem's
suburbs, Ramot Polin – "a housing project for
honeybees":
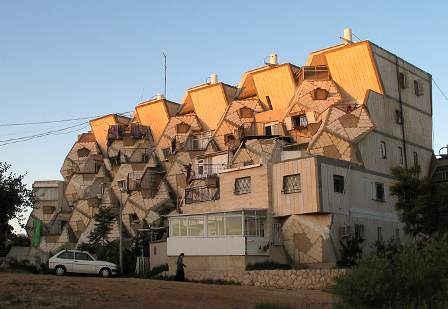
This is consistent with the style guide, but not very consistent with the actual look of other buildings –
nor does it look very comfortable. Leading to my last observation:
- A common style can be codified and enforced, but common sense can't be. Municipal law mentioned
"off-white", but who would have thought to mention "rectangular"?
A sensible style guide is your one and only way to achieve consistent style – and not much else.
What if a style guide is not sensible? Here's a building from Tirana, Albania:
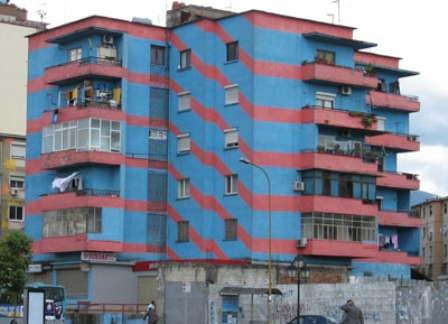
Here's another one:
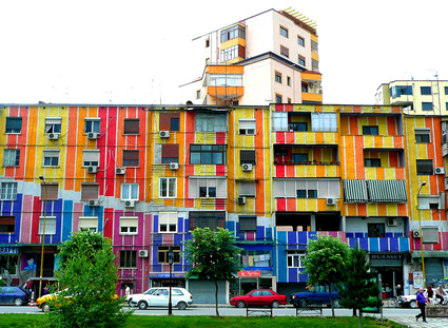
Yep, that's the style guide over there – bright colors over ugly buildings. And there's nowhere to hide from the consistent
style.
Maybe you actually love this style, and hate Jerusalem's uniform off-white. My point is that either way, the consistent style
of one of those cities leaves no place for you to like.
Tel Aviv, on the other hand, has a place to like for both Tirana lovers and Jerusalem lovers. Off-white houses with red
rooftops? Neve Tzedek has what you want:
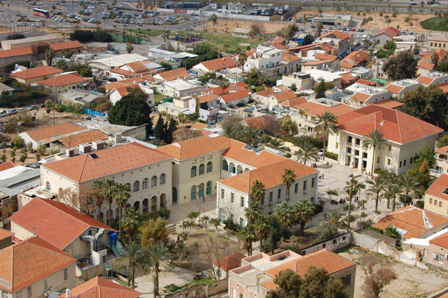
Buildings painted in primary colors? Here's a hotel for you:
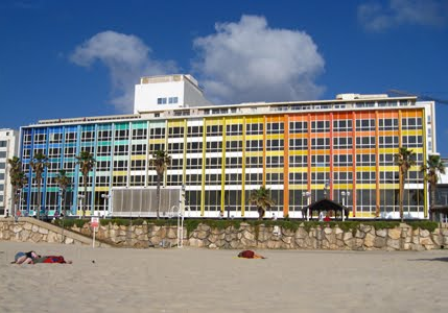
Personally, I still prefer Jerusalem though. Consistent style is better – if you like that particular style.
Requiring limestone vs banning asbestos
Can coding standards be described as style guides, or are they more than that?
The Google C++ Style Guide suggests that it is
in fact more than a style guide:
The term Style is a bit of a misnomer, since these conventions cover far more than just source file formatting.
The document goes on to say that, apart from "enforcing consistency", it also "constrains, or even bans, use of certain
features" – "to avoid the various common errors and problems that these features can cause".
"Enforcing consistency" does sound similar to requiring limestone – there's no direct functional impact. But "banning
features to avoid problems" sounds more like banning asbestos – very much because of its functional impact, which can include cancer.
However, language features are different from building materials. Asbestos was discovered, not designed, and they couldn't
know it'd cause cancer. C++ RTTI was designed and approved as a standard by strong programmers, who had in mind some cases where
they thought it'd be useful.
RTTI is banned by the Google Style Guide, not the way asbestos is banned by regulations, but the way some sculptors prohibit
their students to use fingers when they shape the fine details of clay. Learn to use proper sculpting tools – then do
use fingers if necessary:
A query of type during run-time typically means a design problem. ...you may use RTTI. But think twice about it. :-) Then
think twice again.
Think four times and you'll be allowed to use RTTI. Think 1024 times and you're still not allowed to use asbestos in a
housing project. That's because construction standards include functional considerations, but coding standards ultimately
discuss style and style alone.
That's why the strictest coding standards allow exceptions. And that's why every banned feature is sometimes better than the
proposed alternative.
Readability through inconsistency
Style guides enforce consistency. In the real world, we've seen that consistent style matters psychologically. In
programming, people also advocate consistency for psychological reasons:
It is very important that any programmer be able to look at another's code and quickly understand it. Creating common,
required idioms and patterns makes code much easier to understand.
Psychological reasons are important – but there are symmetric psychological arguments for inconsistency.
For example, required idioms can in fact make code easier to understand – or harder. Let's look at idioms actually required
in some style guides:
- The use of C++ "algorithms" such as std::for_each and std::transform instead of decade-old "patterns" called loops. I expect
the idea to become widespread again, together with C++11 lambdas. Here's TheRegister's take on the impact on
readability.
- Yoda conditions: if(5 == num). This page –
first Google hit for "Yoda conditions" at the time of writing – lists only benefits and no drawbacks, and proposes to add this
to your style guide. Will code become more readable though? They're Yoda conditions! "If num is five" is how you always
say it in English (and Hebrew, and Russian). If five is num, read as natural your code will not.
Of course my opinion on the readability of these patterns is debatable – which is precisely my point. Once a style guide is
chosen, some people will experience the joy of fluent reading every time they hit if(5==num). Others will experience the pain of
a mental roadblock – also every time.
A style guide will have something to dislike for everyone. When tastes are sufficiently different, the average amount
of cringes per person stays the same under consistent style – and the variance rises (someone will
hate a particularly popular mandatory pattern).
It's like keeping wealth constant and increasing inequality – something not even a political party would advocate. How is
this psychologically a win?
But let's go ahead and assume that the "required idioms" suit everyone's taste, and, by themselves, actually make code easier
to understand. Still:
- External libraries will not follow your style guide. They follow style guides of their own. And this
inconsistency can improve readability. Code using the library stands out, and the library's style can match the
accepted domain-specific conventions better than your local style. In computer vision, X is the real world coordinate, x is the
pixel coordinate – contrary to many software style guides.
- You can't count on stylistic conventions, because there are exceptions. Google's code orders parameters
such that inputs come
first, but memcpy & snprintf don't. You either have to look out for exceptions or risk misunderstandings by blindly
assuming consistency.
- Different people think differently, even if their code looks the same. I find it easier to understand
programmers' intents through their unique style. When they're all forced to write superficially similarly, I can't tell who
wrote what, and what the subtext of the code is.
I'll illustrate the last point with a couple of examples. I knew O.M. before I ever saw him and before I even knew his name.
To me, he was the programmer with the two spaces before the trailing const:
inline int x() const;
I knew him through his code: mathematically elegant, obsessive about fine details of type-based binding and modeling. I could
guess what he left out with an intent to maybe add it later. I understood him.
Likewise, I can always spot G.D.'s code by the right-leaning asterisk:
int *p,*q=arr+i;
G.D. certainly couldn't care less about types – similarly to most people with this asterisk alignment. I know his code:
terse, efficient, to the point. I know what to expect.
Who wrote this code?
camelCaseName = longerCamelCaseName-camelCaseName;
I dunno, the collective unconscious wrote it. Anyone could write it – or several people patching after each other. I fail to
identify with the author and guess his intent – it could be anyone. The code has no smell or taste to me.
This can sound a bit crazy – "does he actually advocate that everyone develops as uncommon style as possible as a
way to mark his trail"? Of course I don't mean that.
I think what I'm saying can make more sense if you think of style and taste in code as analogous to the taste of food. Of
course it's ridiculous to expect every restaurant to make food with a unique taste. Many people like pizza, many people know how
to make pizza – so expect much similarly-tasting pizza around.
But we wouldn't like to always eat food cooked to the same spec by restaurants in a single franchise. If someone knows to
make food with a unique taste, we welcome it.
And unique taste of your food doesn't indicate that it's bad for your health. Moreover, food with a familiar taste can be
very unhealthy – much of it is.  Code in a familiar style has a comforting look – sometimes misleadingly.
I don't think requiring the same taste everywhere is how you improve the health of a code base.
Popular demand
I'm naturally inclined to argue against coding standards, because it feels like bureaucracy unthinkingly imposed on the work
process from above. However, what if it's not imposed from above – what if the programmers themselves want it?
Many certainly do. Many good ones do.
Incidentally, while I was writing this, I stumbled upon an article arguing for consistency – for the
very reasons I use to argue against it:
If code isn’t written in a consistent style in your team, whenever you come across code with the spacing a bit wrong, the
first thing your head’s going to process is "I didn’t write this."
...Exactly why I like personal style! I really didn't write it – it's his code, I want to
understand him, and his style helps greatly.
This is a natural feeling, and as we all know coders have a hard [time] to restrain impulse to rewrite any piece of code they
didn’t write.
I understand that impulse very well. Personally, I hate new food more than anyone I know – I eat the same thing every day,
for months, for years. I do prefer Jerusalem to Tel Aviv. And I like consistent style – especially my own.
But why should others be forced-fed my favorite cabbage salad? Is consistency that much prettier than freedom?
Team spirit
I don't argue with people who favor a consistent style – and I can't. The article above is nostalgic about a team that
followed a style guide "religiously". How can nostalgia be refuted? Clearly, consistent style can create a unique team spirit
that to some is valuable and memorable.
All I can and do argue is that a lack of a style guide can also create a good atmosphere that is preferred
by some other people.
In fact I believe it is exactly team spirit that a style guide – or lack thereof – actually affects. All other effects come
indirectly through the impact on atmosphere. Here's my attempt at taking the social effect into account:
- I won't break established conventions. Following conventions is a great way to say, "I respect the local
tradition and the wisdom it embodies." And I'll thoroughly enjoy the limestone covering our code – I like consistency.
Yay, a beautiful code base! And if the convention is really bad – I hopefully won't need to join the team in the first
place.
- However, I won't establish and enforce conventions when I'm the one starting with a clean slate. Having no
conventions is a great way to say, "join my project to express yourself without artificial constraints".
From team spirit to grassroots bureaucracy
Did you know Ken Thompson is not allowed to check in code at Google? He said so in his Coders at Work interview:
Seibel: I know Google has a policy where every new employee has to get checked out on languages before
they're allowed to check code in. Which means you had to get checked out on C.
Thompson: Yeah, I haven't been.
Seibel: You haven't been! You're not allowed to check in code?
Thompson: I'm not allowed to check in code, no.
The programmer who co-created Unix is not allowed to check in code. If this isn't a bureaucracy, what is? But it's inevitable
– with rules, you always paint yourself into a corner that way. Allow some to break the conventions, and you will have offended
everyone else. Use the same rules for everyone – and some won't contribute.
Of course, Thompson does contribute to Google. Well, Google has plenty of ways to motivate him to do that. And, apparently,
they have good programmers willing to write production code based on his uncommitted prototype code.
But we're not all like Google that way. We can't all afford the inevitable laughable outcomes of bureaucracies. If you come
across an original programmer with an off-beat style, do you want him to join your project or to move on?
Grassroots bureaucracy is still bureaucracy. I wouldn't object to an established bureaucracy that people claim to value. But
I wouldn't establish a new one, either.
Conclusion
A style guide can make code look prettier to some – and uglier to others – but not tangibly better, except if
programmers enjoy it so much as to produce better code.
This is equally true for the lack of a style guide. The results of freedom look prettier to some and uglier to others.
Personally, I believe that one rule too few is better than one rule too many, so I don't bother to enforce a common
style.
P.S.: when I become a neat-freak
Generally, I tend to enforce little to no
conventions. Here are the exceptional situations where I actually enter the ridiculous position of telling people how to
write their code.
Interfaces should be consistent
I don't care if an interface looksLikeThis or like_this. But if it uses both, then I'll ask the author to change it
to one of the styles – the one which came first. For users to feel confident, an interface should look well thought-out – which
implies an illusion of a single author, which implies a consistent style.
By "interface", I only mean the outermost stuff called by module users. Internal functions, classes, etc. can look like
Tel-Aviv as far as I'm concerned. For instance, in a simple server, the "interface" to me is just the protocol, and nothing in
the code itself.
Warnings should be errors
I hate the concept of compiler warnings – I link it to the concept of guilt. "Fine, be that way, do this evil implicit
conversion thing – but if something happens, I will have told you so." Why should we put up with such manipulative behavior?
Pick a position – refuse to compile, or compile silently.
However, in practice, compatibility issues make what has to be errors into warnings. If it compiled 30 years ago, it has to
keep compiling, even if nobody wants it to compile in new code. Even if compilers could always prove the code wrong, but
initially didn't bother, and it happens to work in old programs.
So, to the dismay of freedom-lovers, I turn warnings into errors where I can (as in -Werror) – even if I can't cherry-pick
the "right" warnings. Mainly since when a file generates 10 (false) warnings, the eleventh (truly useful) warning goes
unnoticed.
Another reason is that warnings cause guilt – they are evil, and must be destroyed. If I didn't destroy them by turning them
into errors, I'd have to destroy them by disabling them. Then I'd never get that eleventh useful warning.
But except for these two pet peeves – interfaces and warnings – I do think grown-up programmers should be left alone.
Originally, I had a few references to Bauhaus architecture in the text – how Tel Aviv has buildings in the Bauhaus style and
Jerusalem doesn't because of its limestone requirement, and how Ken Thompson's inability to commit code at Google is analogous
to that. However, as a commenter pointed out, there are buildings in the Bauhaus style in Jerusalem – in my own
neighborhood, actually, so I obviously walked past them plenty of times.
I guess this goes to show that good architects have no trouble complying with a style guide – and that I shouldn't overextend
metaphors in areas where I'm not minimally competent.
It can be even worse. I can barely tolerate *reading* the Mono source
code because of their convention of putting a space between a function
and the opening parenthesis of the arguments, so you get code like "foo
(x, bar (y, z))", it just looks so hideous my eyes almost bleed.
Needless to mention, I could never contribute to a codebase so
afflicted.
There are two aspects here to focus on regarding coding styles, and
also relation to real world architecture!
You will find this is repeated around the world and is a very common
pattern. For a housing market or land market, that is highly regulated
the more expensive it is (typical housing bubbles develop). Though with
added consistency and psychological pleasing architecture comes at a
price, and that is the barrier to enter is higher, and thus natural
monopole start developing.
The second part, you've were talking about is style or common pattern
when reading source code. I've been wondering about this for some time,
and why this is the case. What fundamentals reasons could be there for
programmers to fight so much over this over and over again. I've seen
programmers fight in the office regarding style, and having a consistent
pattern. The management comes along and says that everyone should follow
a define style to stop people for a better or less wasting time.
The trust is..... at a funder-mental level IT MATTERS, it MATTERS a
lot.
It's not about style, it's not about being pleasing. It goes deeper
than your frontal cortex and your eyes.
The full picture can be found by reading the book from (bill hill)
"The magic of reading" on his blog, its a free document about how we
read.
Here is the link.
http://billhillsblog.blogspot.com/2008/02/magic-of-reading.html
For a quick summary of the article. The human eye has a resolution of
0.2mm thick. This only gives us a very narrow resolution when we read.
So this means, that the best font sizes to read source code is 10 to 12
point in size, anything above and bellow this the speed at which we read
drastically falls off.
We've evolved from hunters and gathers species that inherently
pattern matching was key part of our survival. Being able to quickly
identifiy friend and foe could mean the difference between survial and
also death. So, we all have these traits from the cave man days. Reading
a book or text is using these same fundermental skills of reading on the
computer screen. So font that are serif and are 10 to 12 point in size
are inheritently faster to interpret than font's that aren't.
So why are all the programmers fighting and will allways fight over
style?
Because they're not fighting over style, or what looks good. They're
fighting over a fundermental lower pattern reconition. For them, their
style takes no concious effert to read. So they can instinictly can read
their code un-conciously. This allow's them to focus their mind at the
algorithems and the data structures like hunting a animal in the
wild.
When something changes, or pattern changes they lose the ability to
un-conciously read the text and have to mentally conciously focus on the
text on the screen to interpret what is going on. This is where the
problem is and everything that is talked about it is all a red
herring.
Programmers are not fighting over style, aritechture or beauty,
they're infact fighting over the ability to read on the screen at the
most optional rate, and this is typically naturally related to
un-concious reading).
The reason why coding standards are used, and typically miss the
point COMPLETLY. Is that it allow's everyone to agree on a standard, but
more fundermental than that, it allows all developers to move from
concious reading to un-concious reading of the source code. It works, it
creates a huge amount of fighting, because no-one has the same tracking
and pattern reconition for hunting.
As a conclusion of all this, I agree with you with your blog about
the style, though at a fundermental issue everyone is talking like we're
these supreme beings and we're human 2.0 when in-fact, we're primitive
human 1.0 version. Sooner we accept it, and adapt our working practices
a lot of these problems will go away.
@Barry Kelly: I guess I could live with the space after the function
name. Would you not contribute, in theory, even if much of the code used
that style, but some wouldn't, and you wouldn't have to put that space
in your own code?
@Entity: It's an interesting perspective, but the whole point of
vision and the reason it pays to carry around so much brain tissue to
support it is that it's my problem to recognize what I see –
not a problem of the things I see. Coders forcing their style on others
are like hunters demanding prey to have a standard look – exactly the
opposite of what prey wants. If prey wanted to be seen, it'd transmit a
unique frequency and we'd have a receiver for it – much more economical
than vision.
True, fellow coders are supposedly your friends as opposed to hunted
animals who sort of have the opposite agenda from yours. On the other
hand, we can't create a single coding monoculture just because there are
too many programmers to coordinate their style. Therefore you'll
necessarily have to deal with code not following your preferred style –
and I'd expect vision, which was designed to cope with adversarial
strategies with regards to appearance, to be able to deal with
non-adversarial, "just different" patterns introduced by other humans.
Certainly my vision prefers inconsistency in a way.
Keep in mind newcomers to a project can be demanding for coding
conventions.
Following conventions makes them confident about their work being
accepted/integrated into the code base. They're even more at ease if
they know they can bend rules if they really want/need to.
But since all people in the project are supposedly equally smart,
they just accept to adapt themselves, cut endless debates and move on
doing interesting things
There is, of course, a personality behind every coding style.
Sometimes it's an interesting one, sometimes unkempt, sometimes somewhat
boring.
Humans are adaptable beings. That's why they survive. You can adapt
to a coding style. I did this many times. It takes some effort and at
first you have to concentrate a bit harder to read the code. Obviously,
the less you need to switch the style, the less effort you spend.
The worst case is when everybody is using their own style and
everybody need to change everyone else's code. It is more effective to
switch the style once than to switch it every day, even if *everyone*
needs to switch their style.
It is often not possible to maintain a single style, especially in
big projects: different languages may be used, there could be
administrative boundaries, third party libraries, some style suits some
problems better, etc. A whole other can of worms is the personal
aesthetics.
So, you should strike for balance, but less varied style is more
effective.
All those talks about coding standards... I just don’t get it. From
my experience (which is not so solid at this point), coding standards
are important on a much higher level – on the level of loose
coupling/high cohesion, design patterns, etc. Vars and class and method
names – this is from another opera.
All homes in Jerusalem and in Tel Aviv are designed with loose
coupling/high cohesion in mind, they are separate and have well-defined
simple interfaces (doors, windows, address (which is not a completely
unique id but still..)). Anyone from another country can use those
simple interfaces very well. So why bother with roofs color?..
If you arrive in a city and you see that (its written in perl haha)
it has actually one big house instead of separate houses, and you cant
get inside, cause it does not implement EnterenceAndWindows interface,
or the door is 7 kilometers above the sea level... Would you be much
concerned about the fact that this houses walls are painted with
green?
Coding standards can somehow help you program faster, or to keep in
mind more things relevant to your project or manipulate them with more
ease? I don’t think so.
I think its more like the real world – first you know what you are
going to do, then you’ll get the details on how you will do that
(intention first). For example you want to get drunk with Dave – you
surf the documentation on how to, then youll use IDE with autocomlition
to quickly make it happen. Would it be
Humans.CurrentLocation.Dave.Deeds.getReallyDrunk(Me, Today, true);
or it would be more like
humans.current_location.dave.deeds.get_really_drunk(me, today,
true);
or even
thingsToDoNow.getDrunk(Dave, extremely, yes_i_mean_extremely);
Who cares? I surf documentation, use autocomplition and not storing
in my mind method names (even if i did, it would be too easy to mix
things up independently of using coding standards (is it getDrunk(Dave)
or Dave.getDrunk(true) ? I don’t have to know!))
This is brilliant. After all the "This is the PERFECT style, all
others suck!" blog entries, finally we have somebody producing a blog
entry saying something different. And I love your Jerusalem v. Tel Aviv
example.
Why, you've even said code having multiple styles in it is okay! I'm
sure I'm going to be quoting you someday soon.
I have been following a particular style convention that I tweaked
from one of my first post-college jobs.
But mainly, I am a stickler for consistent indentation. This gets me
more than anything else! There are many reasons, but one is that when I
am working on a section of code, I usually work directly at the left
margin. Code-in-progress sticks out like a sore thumb.
I also hate inconsistent indenting in general, and it is practically
universal. What is so hard about doing it right?!
No Bauhaus in Jerusalem? You should take a look at http://artlog.co.il/jerusalem/, or just walk around
Rechavia.
That code consistency is merely a question of style is a very common
misconception. It assumes that the role of the code is solely to make a
computer work and ignores the second important function: communicating
with other programmers. I'm glad that others before me already pointed
this out.
If we want to use the building analogy, we have to mention that
architects are significantly less creative and much more cautious when
designing building features people actually use. Randomly placing steps,
doors, interior walls, or elevator buttons doesn't earn you better
reputation in that trade than writing messy code earns in ours. Your
building analogy mainly considers the external, purely esthetic aspects
of buildings, ignoring the functional ones.
It is quite unintuitive, but the fact that we find it mighty
difficult to maintain code consistency in larger projects actually
proves how important consistency is for programmers. Inconsistent
one-man projects are rare. Most of us develop a personal coding style
and stick with it. We become addicted to it to such extent that adopting
a different coding style when we switch projects, organizations, or
programming platforms is quite painful. We try to be consistent, but
consistent with ourselves rather than consistent with our current
project. In other words, we try to minimize our own pain, not the pain
of others. This is quite natural.
People who argue for strict coding standards are extremists just like
people who argue for no coding standards at all. The right approach is
somewhere in the middle, but where in the middle depends on the kind of
the project we are talking about. I personally do API design these days,
an extreme case where we try to make things as painless for the caller
as possible. And yes, we adhere to strict coding standards.
If you are still with me, here is my own blog post on the topic of
(API) consistency:
http://theamiableapi.com/2011/09/14/api-design-best-practice-consistency/
@Roi: I live in Rehavia and walk around plenty; you assume that I'd
recognize Bauhaus architecture when I see it... I'll add the correction
to the text sometime soon...
To all those saying consistent style is better than inconsistent: I
can't argue with you, but you can't argue with me when I say it's worse
:) Specifically, @Ferenz: you say "That code consistency is merely a
question of style is a very common misconception" (because of
"communicating with other programmers" – that concern I understand and I
talked about it elaborately) – then go on to say that "Inconsistent
one-man projects are rare. Most of us develop a personal coding style
and stick with it." – that is, you go on to use "consistency" and
"sticking to a coding style" interchangeably!
I understand the fact that code style helps communicate with other
people (or gets in the way) – that's what I call "psychological
considerations" (the code works either way but can be easier or harder
to follow depending on style). I'm not saying it's not important, just
that "style" is a valid term for this, that it is impossible to resolve
issues of style resorting to arguments on function other than
readability and other psychological, subjective concepts, and that there
are people (for example, me), who prefer to follow code in many
internally consistent, personal styles rather than a code base as if
written by the Borg where you have no idea who wrote what and where
inevitable personal differences in thinking are masked by unity of
visual style.
The upshot is that you can't argue what makes communication easier
for me anymore than I can argue what makes communication easier for you!
The "objective" measures are opinion polls (haven't seen any and those
are problematic because of selection bias – people who don't care about
style are unlikely to care about polls), and measuring productivity
under the alternative regimes (but we can't measure programming
productivity). So this sort of has to remain subjective, and therefore,
I believe we're all entitled to our opinions, as long as we state them
as truths about ourselves and not the programming population at
large.
The biggest problem I've seen is people making the standards are
usually very senior programmers and tech changes relatively fast, so
when programming C++ I had to deal with cruft from C, Pro C, Ada and god
knows what else. Then when using java I had to deal with the leftovers
from C and C++.
My feelings are mostly the same, though. If you have to explain the
drawbacks of RTTI or extern or goto then maybe you just don't need that
programmer in the first place. But when you codify it you burn into
people's brains something is bad. People seldom use pure virtual in C++
where it's appropriate, and gotos exist for very good reason. And no
matter how big a list you come up with it's the horribly unexpected
thing that will cause you trouble.
@Yossi If my comment sounded like I missed the finer points of your
post, I'm sorry. I would not have bothered to comment if I completely
disagreed with you. And yes, I used some words interchangeably and
inconsistently... quite annoying, isn't it?
Well, it wasn't annoying at all, just showed that you categorize
concerns regarding consistency as "style" yourself :)
BTW, I agree that APIs should be consistent and I made an explicit
exception for them in the post. In an API-intensive effort like the one
you mentioned, sure there has to be a person in charge of consistency. I
just think it isn't a big win in implementation code, and most of the
code is implementation code.
The honest truth about code styles, is that your brain can get used
to pretty much anything, if you just force yourself to use it for two
weeks. I used to *hate* the K&R brace style, then I found myself on
a team that enforced it, now I actually prefer it.
But, your brain never quite gets accustomed to inconsistency, so if
your code has a mix of styles then those subtle differences will always
be a distraction. If your ultimate goal is to solve a real problem
(rather than personal expression) then you want to discourage those
distractions.
As far as recognizing the author by code style, this is another bad
thing I think. It's a bad code smell to have code that is "owned" by one
person; this tends to discourage other people from reading or modifying
the code, and you end up with bugs that only one person knows how to
fix.
Funny that the very first comment vows against the space(s) in 'f
(arg, arg)', as I do it that way (and in an earlier life did
'f(arg,arg)', but seriously cringe at 'f( arg, arg )'. Who did even come
up with the latter, anyway?
I don't think buildings or food are good analogies for code when
discussing style and standards.
I think of code in terms of communication between two or more people,
rather than communication between people and machines. When writing, one
should consider their audience. Just as I wouldn't put "ROFLMAO" in a
legal contract, I am not likely to use "heretofore" in a text to my
son.
@Andy: you can tell me what your brain gets accustomed to and what it
doesn't get accustomed to; you can't really tell me about mine :) As to
code ownership – I actually quite like it: http://www.yosefk.com/blog/extreme-programming-explained.html
@Michael: I don't edit legal contracts for a reason... As to code –
sure, it's communication between people, and we do have to consider the
audience, but does this mean that all writers or public speakers or just
people talking to each other should all use the same style? To continue
your analogy, I believe that a good editor leaves the author's style
alone, but can give a good advice on making the overall structure of the
text more comprehensible – and proofreading is handy, too. Then there
are editors who nitpick and won't leave any of your sentences alone –
those IMO are bad editors, and I believe that very strictly enforcing
style in code comes from the same instincts.
I had a conversation once about how the w.c. pan cover to be treated
in the optimal way – should you being man (in terms of standing peeing)
or woman (in terms of sitting peeing) how the pan cover to be left – up
or down and how it depends on the sexual being of a previous visitor.
And my claim was the optimum can be achieved if you leave the cover as
you found it – once you had it closed entering the lavatory – leave it
closed, was it opened – open it as you leave. The same holds for the
code – once you are entering some others pan – behave like he/she used
to and leave the code in exactly same conditions like it was before.
Just do not touch the internals...
@Efim: that's what I do myself, usually. However, when someone
changes my own code, sometimes I like the fact that the change looks
like someone else's code – easier to spot. If someone is behaving "like
me", he better actually behaved like I would – if he's emulating my
style but does things very differently from the way I'd do, it's
actually harder to figure out what's going on once I no longer remember
my own original code enough to easily tell which parts are my own and
which parts are someone else's.
Basically there are two extremes – many people working as one person
and everyone working as himself – both are OK, the latter is somewhat
worse but easier to achieve; and then there's that bad point in the
middle where everyone pretends to be the same person but only succeeds
as far as appearance goes, but not at the level of substance.
(If you insist on discussing it in the terms of your analogy, I guess
that would be leaving the seat down having peed on it without cleaning
it up – because the previous person left the seat down. I believe
whoever enters next would rather have you break the convention and leave
the seat up to having it down according to the convention but peed on.
In code, not everybody is sophisticated enough to correctly pee in a
style foreign to him; I prefer them to pee in their own style but in a
substantially sound way...)
Well, this is just to say hello, was an interesting read ....)
...I recall, BTW, that you once had a machine named tirana.
Back in the early 90s, one of the developers I worked wrote his own
web browser because he didn't like how ugly most sites were and thought
that got in the way of comprehension. His browser put the user at the
forefront, and trying to render things in a way that made sense to them:
better fonts, minimizing graphical content, allowing the user to
override most of the structural junk. (This was the early 90s.) His
point was that no matter what the HTML author wrote, the user should be
able to view it in a way that makes sense to THEM.
I wonder if the same approach can't be applied to code. Why can't
editors show me code in the way that makes sense to ME? If I want
consistent formatting and braces on their own line, make it look that
way. The raw code itself is just symbols and whitespace–presentation
isn't the same as the raw data. Each developer should be able to see
what their brain is used to, without forcing that on everyone else.
I think HTML is a great example of how hard it really is to separate
content from presentation. I think that trying such separation with code
is interesting – "Intentional Programming" (ex-Microsoft) is I think one
of the most developed systems done using this approach, and then we have
Subtext/Coherence – but there are IMO good reasons to all successful
programming languages always using text that is viewed and edited
straightforwardly as text, with a sprinkle of tooltips and
autocompletion.
This is why I use the console and a text only browser to read code
and HTML.
Everything looks more or less the same.
HTML formatting becomes more or less "standardised". Every HTML page
looks very much the same. Only the text is different.
And font variations are eliminated.
Easier reading.
I think I generally agree with Yossi on this: that coding standards
can be good for a project, because it helps the developers working on
homogeneous code, but being able to recognise which individual wrote
what can sometimes be useful, and even important, too.
On the other hand, one idea I came across a while ago (and I
unfortunately cannot find the original blogpost that made this point) is
the idea that certain coding standards should simply be enforced by the
compiler. The person suggesting this proposed that K&R style, for
example, should be enforced for C.
I can see the point of this–it ends the Style Wars, among other
things–but I also suspect that no matter how tightly you enforce
standards, there's always going to be enough wiggle-room to do things
that will drive other programmers batty.
But then, I can also imagine a compiler that enforces a "no space
after a function but before the parenthesis" rule driving a developer
batty, who just spent hours trying to find the syntax error, because he
accidentally placed a space between a function and its opening
parenthesis. The nice thing about deviating from style *guidelines* is
that if you depart from them, even accidentally, working code still
compiles. You could always fix style problems later, and usually, it's
just a cosmetic fix anyway...at least, if it's a syntax thing, it's
cosmetic.
From using Python, though, one conclusion I've made is that enforcing
indentation is a good idea (even when I've occasionally wished I could
add an extra indent to a block of related lines of code, to help it
stand out, and Python wouldn't let me do it).
Yossi, I've worked on a project where one of the coders was using
Whitesmith style, another wa using GNU style, and the the rest were
using K&R style...
Switching between these
if (condition1) {
if (condition2) {
}
}
if (condition1)
{
if (condition2)
{
}
}
if (condition1)
{
if (condition2)
{
}
}
is an outright nightmare both for reading (WTH does this brace
close??) and for writing (your editor won't help you at all with
indentation).
And as code develops/gets refactored and new developers come in, the
islands of consistency shrink, until you have no consistency even at
file level (and it's all downhill from there).
I really *love* R.B.'s idea in post #23.
If you could tell your editor what is the style *you* like so it
renders the code in *your* screen the way *you* like it, then all these
style wars would be over.
@Joe Max: I think it's awfully hard though, as it is very hard to
present a complex website more neatly by taking the contents and using
different styling; works in some cases, works worse in other cases.
With code, specifically, it could be even worse than HTML. What would
you do about longNamesSuchAsThisOne, for instance – how do you show them
to me if I like shortnames? If someone likes to use std::for_each, would
you present it to me as a plain for loop?
The best blog of the year.
I'll just add that a lot of conventions are imposed because of
limitations of tools, notably revision control systems and the diff
algorithm.
But we could easily enough set up an IDE (eg. emacs), to unparse an
internal representation (sexps) stored in files and revision control
systems, and to produce a source text in the prefered style of the user.
When the file is saved, it is parsed and saved back into a normalized
form, that is never read by human readers, but only by the tools (diff,
compilers, etc).
Thanks! As to non-textual representations of source code – I'm
guessing the reason it wasn't ever done successfully (though MS did it
once in a never-adopted product) is that it's harder in practice than in
theory.
I actually prefer no coding style. Because a coding style will
distract many programmers when reviewing other people code. for example
they will flag code because of the style and not because of an error in
its content.
for example the programmer wrote checking a pointer if it was
valid:
if(something)
but to check if it is invalid
if(!somthing)
The reviewer might flag this line of code because the styling guide said
has to written like:
if(something != nullptr)
But the reviewer misses the obvious error.
So every team should use an auto formatter, does away with the human
linter like reviewing and gives the added bonus that
that refactoring with regular expressions becomes trivial.
Thus the style guide should only include things that can be enforced by
a code formatter.
Also if someone prefers a different style he can just use the auto
formatter to convert to a different style.
Also when people read the same style then it becomes hard for them to
read in a different style.
They will think less about the code they read and over look most of the
errors.
Something relevant but not directly on code:
https://www.youtube.com/watch?v=cebFWOlx848
But what really nags me are coding conventions that make you code
verbose, obscure or are for the sake of readability.
for example
-you have to put all constants at the top of the file.
-you are not allowed to use the conditional operator.
-do not use ! but explicitly check with == 0 or nullptr etc.
A convention should help programmers avoid common pitfals or hacks
Like.
- don't use exceptions for the program flow
- friend objects should be avoided.
- use override for an inherited virtual function.
But not :
- lamda function should not be longer than x lines.
- std bind is not allowed.
Even worse are coding conventions that ban features or only allow a
small subset
of the language just because of the sake of readability since that is
highly subjective.
like:
- don't use typedef,
- don't use higher order functions.
- don't use anything that is not OOP.
All these rules reduce flexibility and thus make code less reusable
and maintainable. Or straightforward to use.
For me a better rule would be a library should trade in readability
and complexity for flexibility.
Thus making the application code more readable and simpler and
shorter.
My experience has been that nonsensical rules are always pushed
hardest by the least capable developers.
They can't read other peoples' code. Instead of improving their
reading skills, they move to handcuff everybody else into their typing
pattern. What's ironic is that doing this ignores the possibility that
other developers can't read their code either. Now those developers have
a second problem, trying to write code that they already couldn't
read.
What's worse, poor writing usually follows from poor reading. The
readability police rarely follow their own rules. That, to me, suggests
that the problem isn't in reading other developers code but reading code
in general. Forget the question of why an illiterate gets to dictate
spelling and grammar, why do we have somebody who can't read or write
code employed as a developer at all?
Small wonder that so much software these days is barely functional
and full of holes...
Post a comment